Creating custom behaviors |
A Behavior can act without any external notification from a Trigger or a Trigger-like item. While some condition has to be met, it doesn't have to be invoked by a Trigger.
Simple Behaviors
Writing a Behavior that requires no external input is fairly straightforward. The following code sample shows a baseline Behavior:
C#� | ![]() |
---|---|
public class MyBehavior : Behavior<DependencyObject> { public MyBehavior() { } protected override void OnAttached() { base.OnAttached(); // Insert code that you want to run when the Behavior is attached to an object. } protected override void OnDetaching() { base.OnDetaching(); } } |
Extend the Behavior class and constrain it to the type that you want your Behavior to be associated with.
Just as you can with OnAttaching and OnDetaching methods for Triggers, you can put any code that you want to run when the Behavior is either associated with an object or is being disassociated from its currently associated object in the OnAttached and OnDetaching methods for Behaviors.
Behaviors with Commands
One way to allow users to customize the functionality of your Behavior is to expose Commands (any property of type ICommand), each of which corresponds to an action the Behavior can perform. First, make sure that your project has a reference to both the Microsoft.Expression.Interactions and System.Windows.Interactivity DLLs. Next, create a new Class file, and add the following code:
C#� | ![]() |
---|---|
public class BehaviorWithCommand : Behavior<DependencyObject> { public BehaviorWithCommand() { this.MyCommand = new ActionCommand(this.MyFunction); } protected override void OnAttached() { base.OnAttached(); } protected override void OnDetaching() { base.OnDetaching(); } public ICommand MyCommand { get; private set; } private void MyFunction() { // Code to execute when your Command is called } } |
The following diagram provides an overview of how Commands and Behaviors work together to give you Action-like capabilities:
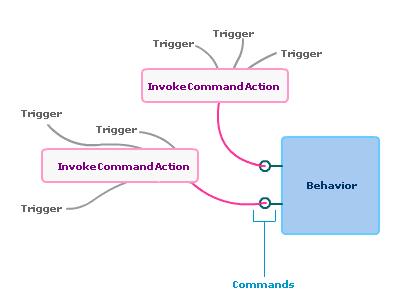
As shown in the preceding diagram, a Behavior exposes Commands. The existing pattern of Triggers and Actions can be used to invoke these Commands by adding Triggers to your Behavior, and having those Triggers fire one or more InvokeCommandActions. InvokeCommandAction does only one thing: it calls a Command that is exposed on a Behavior.
See Also
��Copyright � 2010 by Microsoft Corporation. All rights reserved.